반응형
이번 문제는 로직이 어렵지는 않은 코드가 더러운(?) 문제였다고 생각합니다.
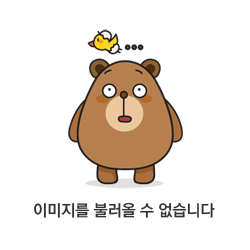
저희가 아는 마방진에서 어떤 숫자가 지워졌을 때 해당 숫자를 구하는 문제로 보고 먼저 한 줄 합을 구하고(빠진 부분이 없는) 빠진 부분의 합에 대입해서 빠진 숫자를 구해야겠다고 생각했습니다. (식으로 하면 X + 12 = 33 이런식이겠죠) 또 3X3 사이즈도 고정이라 하드 코딩이 될 거 같다고 생각했습니다.
먼저 빠진 부분이 없는 줄 합을 구해야하는데 저 같은 경우 처음에 대각선으로 한 줄 합을 구할 수 있는지, 안되면 행, 안되면 열로 구했습니다. 이때 주의할 부분이 예외처리입니다. 저 같은 경우는 이 부분을 고려하지 않아서 실패가 떴고 후에 알아챘는데 바로 대각선이 다 0일 때 경우 입니다. 이 경우 위의 방식으로 줄 합을 구할 수 없기 때문에 방정식을 써서 따로 계산해줘야합니다.
import sys
input = sys.stdin.readline
ma_list = [list(map(int, input().split())) for _ in range(3)]
total_num = 0
# 대각선이 다 0 일때 total 값 구하는 방법
if ma_list[0][0] == 0 and ma_list[1][1] == 0 and ma_list[2][2] == 0:
num1 = ma_list[0][1] + ma_list[0][2] # x
num2 = ma_list[1][0] + ma_list[1][2] # x + (num1-num2)
num3 = ma_list[2][1] + ma_list[2][0] # x + (num1-num3)
diff_num = (num1-num2) + (num1-num3) # 3x + (num1-num2) + (num1-num3)
num1 = num1 - diff_num # 2x = num1 - (num1-num2) + (num1-num3)
num1 = num1//2 # x
total_num = num1 + ma_list[0][1] + ma_list[0][2]
elif ma_list[0][2] == 0 and ma_list[1][1] == 0 and ma_list[2][0] == 0:
num1 = ma_list[0][0] + ma_list[0][1] # x
num2 = ma_list[1][0] + ma_list[1][2] # x + (num1-num2)
num3 = ma_list[2][1] + ma_list[2][2] # x + (num1-num3)
diff_num = (num1-num2) + (num1-num3) # 3x + (num1-num2) + (num1-num3)
num1 = num1 - diff_num # 2x = num1 - (num1-num2) + (num1-num3)
num1 = num1//2 # x
total_num = num1 + ma_list[0][0] + ma_list[0][1]
# 대각선이 다 0 아닐때 total 값 구하기
elif ma_list[0][0] != 0 and ma_list[1][1] != 0 and ma_list[2][2] != 0:
total_num = ma_list[0][0] + ma_list[1][1] + ma_list[2][2]
elif ma_list[0][2] != 0 and ma_list[1][1] != 0 and ma_list[2][0] != 0:
total_num = ma_list[0][2] + ma_list[1][1] + ma_list[2][0]
else:
# 가로 값 확인하기
for i in range(3):
if ma_list[i][0] != 0 and ma_list[i][1] != 0 and ma_list[i][2] != 0:
total_num = ma_list[i][0] + ma_list[i][1] + ma_list[i][2]
break
if total_num == 0:
# 세로 값 확인하기
for i in range(3):
if ma_list[0][i] != 0 and ma_list[1][i] != 0 and ma_list[2][i] != 0:
total_num = ma_list[0][i] + ma_list[1][i] + ma_list[2][i]
break
#print(total_num)
for i in range(3):
for j in range(3):
if ma_list[i][j] == 0:
total1 = 0
if j == 0 and ma_list[i][1] != 0 and ma_list[i][2] != 0:
total1 = ma_list[i][1] + ma_list[i][2]
elif j == 1 and ma_list[i][0] != 0 and ma_list[i][2] != 0:
total1 = ma_list[i][0] + ma_list[i][2]
elif j == 2 and ma_list[i][0] != 0 and ma_list[i][1] != 0:
total1 = ma_list[i][0] + ma_list[i][1]
elif i == 0 and ma_list[1][j] != 0 and ma_list[2][j] != 0:
total1 = ma_list[1][j] + ma_list[2][j]
elif i == 1 and ma_list[0][j] != 0 and ma_list[2][j] != 0:
total1 = ma_list[0][j] + ma_list[2][j]
elif i == 2 and ma_list[0][j] != 0 and ma_list[1][j] != 0:
total1 = ma_list[0][j] + ma_list[1][j]
elif i == 0 and j == 0 and ma_list[1][1] != 0 and ma_list[2][2] != 0:
total1 = ma_list[1][1] + ma_list[2][2]
elif i == 1 and j == 1 and ma_list[0][0] != 0 and ma_list[2][2] != 0:
total1 = ma_list[0][0] + ma_list[2][2]
elif i == 2 and j == 2 and ma_list[1][1] != 0 and ma_list[0][0] != 0:
total1 = ma_list[1][1] + ma_list[0][0]
elif i == 0 and j == 2 and ma_list[1][1] != 0 and ma_list[2][0] != 0:
total1 = ma_list[1][1] + ma_list[2][0]
elif i == 1 and j == 1 and ma_list[0][2] != 0 and ma_list[2][0] != 0:
total1 = ma_list[0][2] + ma_list[2][0]
elif i == 2 and j == 0 and ma_list[1][1] != 0 and ma_list[0][2] != 0:
total1 = ma_list[1][1] + ma_list[0][2]
print(total_num-total1, end=' ')
else:
print(ma_list[i][j], end=' ')
print()
그 밖에는 크게 로직을 짜는데 있어 어렵지 않았던 것 같습니다. 다만 코드가 복잡해서 잘못 입력하면 찾는데 너무 힘들 거 같더라고요 이번 문제는 꼼꼼함을 요하는 문제 아닐까 싶습니다.
좀 더 코드가 간결한 풀이법도 있을지 고민해봐야 할 거 같습니다.
반응형
'알고리즘' 카테고리의 다른 글
[알고리즘/백준] 11052번 카드 구매하기 Python 파이썬 (0) | 2023.04.12 |
---|---|
[알고리즘/백준] 2294번 동전2 Python 파이썬 (1) | 2023.04.10 |
[알고리즘/백준] 2021번 최소 환승 Python 파이썬 (0) | 2023.04.05 |
[알고리즘/백준] 1922번 네트워크 연결 Python 파이썬 (0) | 2023.03.29 |
[알고리즘/백준] 1915번 가장 큰 정사각형 Python 파이썬 (0) | 2023.03.27 |
댓글